人马大战 Python 代码教程:从入门到实战
什么是人马大战?
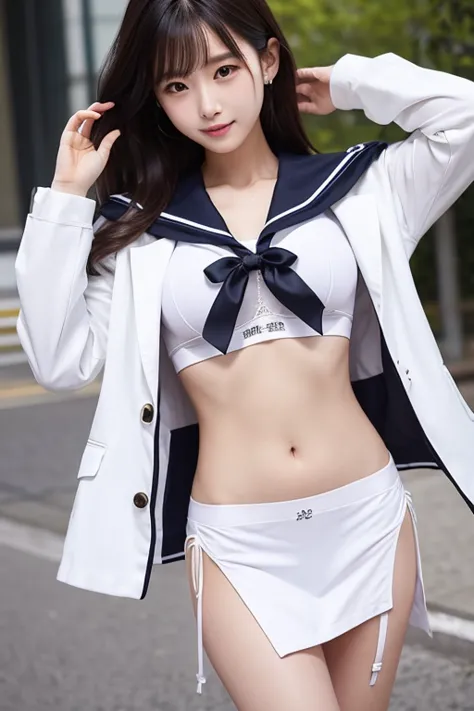
人马大战,是一款经典的策略游戏,玩家需要控制一匹马与敌方的人进行战斗。游戏的目的是通过策略和技巧,击败对手,获得胜利。本教程将详细介绍如何使用Python编写一个人马大战游戏,帮助你从零开始掌握这门编程语言的基础知识。
准备工作
在开始编写代码之前,我们需要确保已经安装了Python解释器和Pygame库。Pygame是一个用于开发2D游戏的Python库,能够帮助我们快速搭建游戏界面和达成游戏逻辑。以下是安装步骤:
- 安装Python:访问Python官方网站(https://www.python.org/),下载并安装最新版本的Python。
- 安装Pygame:打开命令行工具,输入以下命令安装Pygame库:
bash pip install pygame
- 安装代码编辑器:推荐使用Visual Studio Code、PyCharm或Sublime Text等代码编辑器,方便编写和调试代码。
人马大战的核心代码解析
1. 导入必要的库
python
import pygame
import random
import time
2. 初始化游戏
python
pygame.init()
3. 设置游戏窗口
python
window_width = 800
window_height = 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("人马大战")
4. 定义颜色
python
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
5. 定义角色和马匹的属性
```python class Player: def init(self, x, y, width, height, color): self.x = x self.y = y self.width = width self.height = height self.color = color self.health = 100
class Horse: def init(self, x, y, width, height, color): self.x = x self.y = y self.width = width self.height = height self.color = color self.speed = 5 ```
6. 游戏事件解决
python
def handle_events(player, horse):
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player.x -= 10
elif event.key == pygame.K_RIGHT:
player.x += 10
elif event.key == pygame.K_UP:
player.y -= 10
elif event.key == pygame.K_DOWN:
player.y += 10
7. 游戏循环
```python def game_loop(): player = Player(100, 100, 50, 50, blue) horse = Horse(300, 300, 100, 100, green) clock = pygame.time.Clock()
while True:
handle_events(player, horse)
window.fill(white)
pygame.draw.rect(window, player.color, (player.x, player.y, player.width, player.height))
pygame.draw.rect(window, horse.color, (horse.x, horse.y, horse.width, horse.height))
pygame.display.update()
clock.tick(60)
```
8. 碰撞检测
python
def check_collision(player, horse):
if (player.x < horse.x + horse.width and
player.x + player.width > horse.x and
player.y < horse.y + horse.height and
player.y + player.height > horse.y):
return True
return False
9. 得分更新
```python score = 0
def update_score(): global score score += 1 print("Score:", score) ```
10. 游戏结束
python
def game_over():
font = pygame.font.SysFont(None, 74)
text = font.render("Game Over", True, red)
window.blit(text, (window_width/2 - text.get_width()/2, window_height/2 - text.get_height()/2))
pygame.display.update()
time.sleep(2)
pygame.quit()
quit()
人马大战的扩展与优化
1. 添加音效和背景音乐
python
pygame.mixer.music.load("background_music.mp3")
pygame.mixer.music.play(-1)
2. 提升游戏难度
python
class Enemy:
def __init__(self, x, y, width, height, color):
self.x = x
self.y = y
self.width = width
self.height = height
self.color = color
self.speed = 3
3. 添加暂停功能
```python paused = False
def pause_game(): global paused paused = not paused while paused: for event in pygame.event.get(): if event.type == pygame.KEYDOWN: if event.key == pygame.K_p: pause_game() ```
人马大战的常见难关解答
1. 游戏窗口无法显示
- 确保Pygame库已正确安装。
- 检查代码中的窗口设置是否正确。
2. 角色移动卡顿
- 确保游戏循环中的帧率设置合理。
- 优化代码,削减不必要的计算。
3. 碰撞检测不准确
- 检查碰撞检测函数的逻辑是否正确。
- 调整角色和马匹的尺寸,确保检测范围合适。
总结
通过本教程,我们已经了解了如何使用Python编写一个人马大战游戏。从基础的Pygame库安装,到角色和马匹的属性定义,再到游戏事件解决和碰撞检测,每一步都详细解析了代码的功能和达成原理。希望这篇教程能够帮助你快速掌握Python编程的基本知识,并为你的游戏开发之路奠定坚实的基础。